I was talking to a community member about some of the sites I run (and I mentioned a site called https://AppProtection.net) as they didn't realize I also do a lot of server side work. However, she was shocked that the source code for her app was easily accessible by everyone.
If you write an application with NativeScript, React Native, Cordova/PhoneGap/Ionic (and many other platforms) are you aware you ship the entire JavaScript source code, styles, layout code in your app.
For simplicity we are going to look at a NativeScript application that we will generate quickly so you can easily follow along. These steps apply equally for React Native and the Cordova based eco-systems.
You can uses tools like apkeep to download APK's directly from Google play store, or you can use something like Apple Configurator 2 on Macintosh to download the IPA from the Apple iOS store to your computer. Anything published to either store can be retrieved to your computer directly, and that is when the fun begins...
How easy is it?
ns create demo --js cd demo
This command creates a demo application, for simplicity I'm just going to use pure JS, but again this applies to all the NativeScript flavors, Vue, Angular, TypeScript, React, etc.
Now we are going to make some minor changes to the main-page.js file to look like this, as we want emphasize that any secrets are NOT secret! This includes all Tokens, your http(s) URL endpoints, API keys, passwords, usernames, etc....
import { createViewModel } from './main-view-model'; const secret_HTTP_APIKey = "Hi_IM_A_SECRET_TOKEN_USED_ACCESS_SOME_API"; const private_Firebase_Token = "Hi_IM_your_Secret_Firebase_token"; export function onNavigatingTo(args) { const page = args.object doSomethingWith(secret_HTTP_APIKey); doSomethingWith(private_Firebase_Token); page.bindingContext = createViewModel() } function doSomethingWith(value) { <strong><em>console</em></strong>.log("Pretend HTTP Call with token", value); }
Now we will build it:
ns build android --copy-to .
I'm using the <strong>--copy-to .</strong>
so that we copy the final apk to this folder so we don't have to go looking for it in the platforms/android... folder, you should have something like this now...
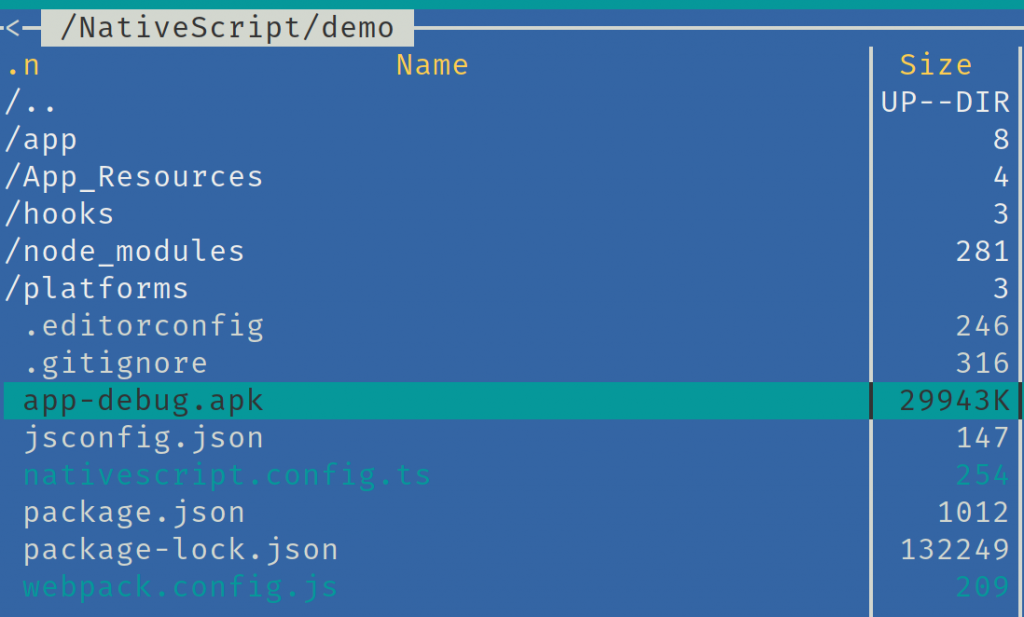
The app-debug.apk
(or .ipa for ios) is basically what is uploaded to Google Play or the Apple iOS store. Lets look at this file. If you unaware a .apk is just a .zip file with a different file extension. In my case, I just renamed it to .zip so that Midnight Commander (my favorite terminal shell) can navigate inside and view files directly....
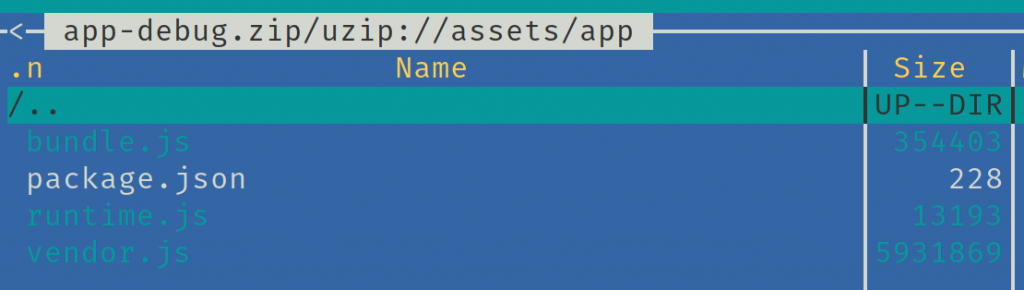
Opening up the .zip/.apk file, and for NativeScript navigating to assets/app you will see the files that matter. The bundle.js is your application code, the vendor.js is all the plugins and NativeScript core code, so 99.9% of the time this is a pointless file for reversing your app. However, if you have custom plugins this would have that code. You can also see any worker javascript files if you are using any workers, and angular has some code splitting that can create some other separate javascript files. The bundle is typically the most important file... Lets just copy it out of the zip file so we can open it into a real editor....
How secure is my JavaScript, lets see the main-page.js?
Opening bundle.js up in PHPStorm, this is what we see:
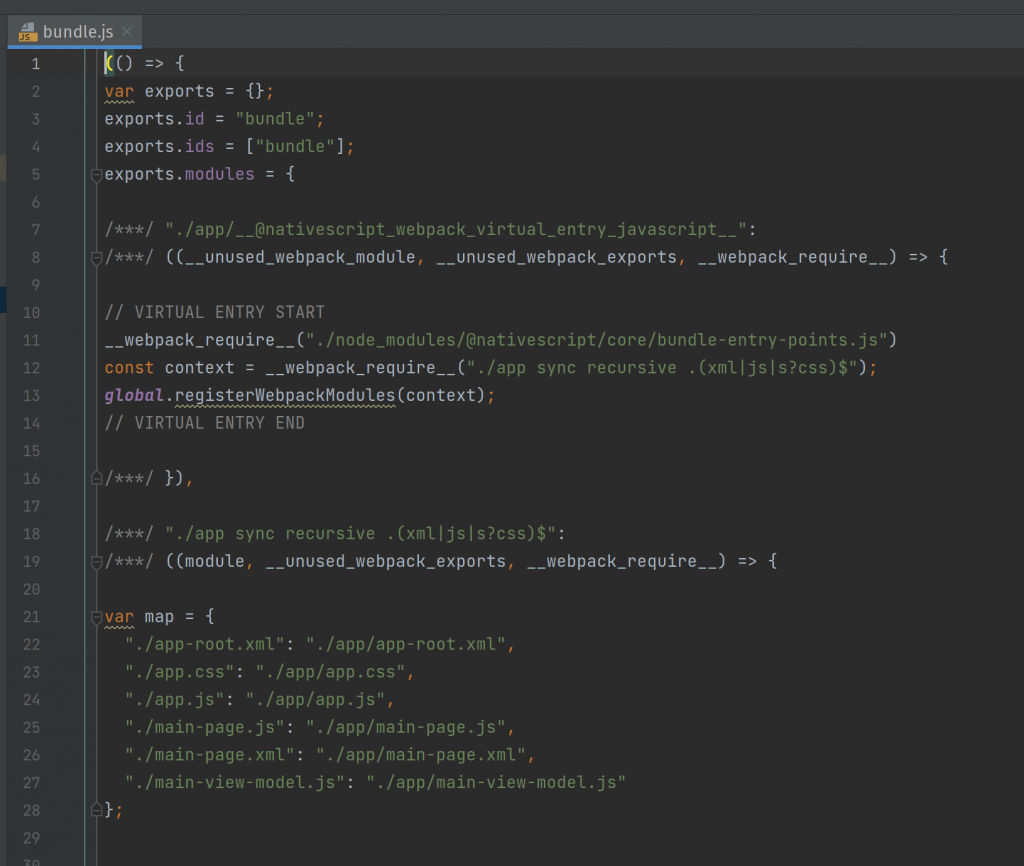
Interesting it tells us we have 6 files that were compiled into this app.
Lets look for the main-page.js inside the bundle! Simply do a search for main-page.js and this what we find around line 100 in the bundle.js file (that is because the prior 80 lines or so is all the CSS that was bundled from app.css and the default imported theme css files)
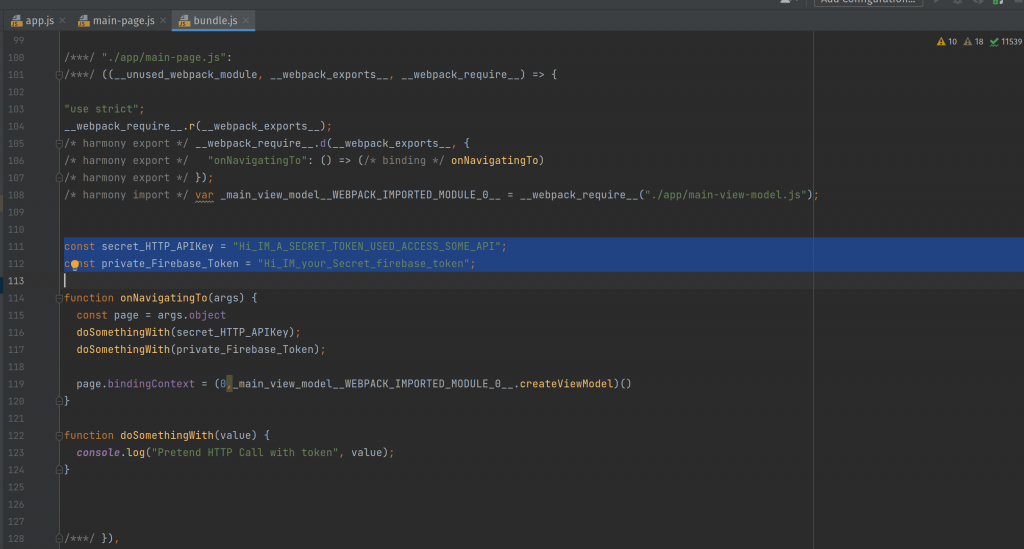
Wow, 100% full source code including all your awesome secret keys and tokens (which I selected)... If you compare this to our file above, the only difference in this code is that we original did <meta http-equiv="content-type" content="text/html; charset=utf-8">
export function onNavigatingTo(args)
and this removed the <strong>export</strong>
from the function on 114 and added it on line 105 - 107 as a __webpack_exports__. Otherwise this code is identical.
What about the layout, Main-Page.xml?
Lets search for main-page.xml, oh it shows up on line 193...
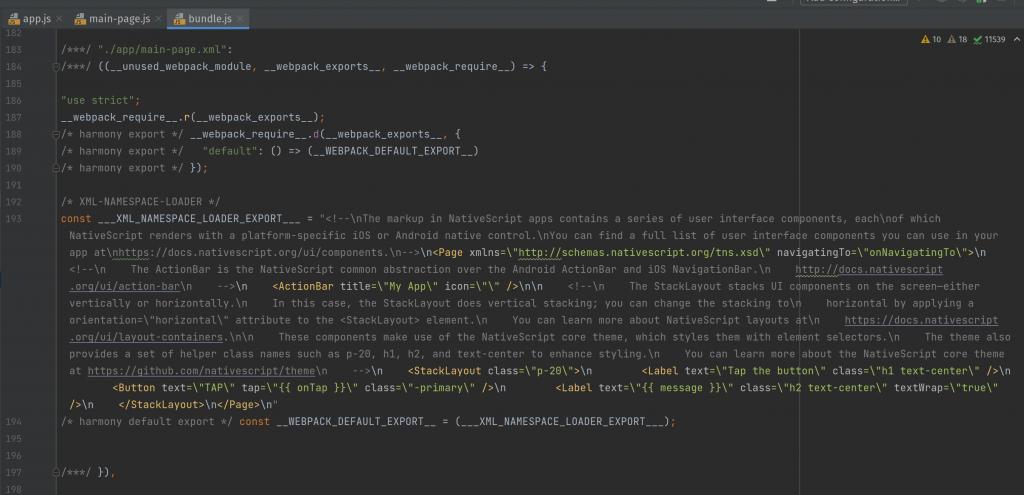
It is just one long string... What happens if we copy & paste it to a newly created test.xml file. How different is this from the original main-page.xml file, not a single change. It is the exact same file.
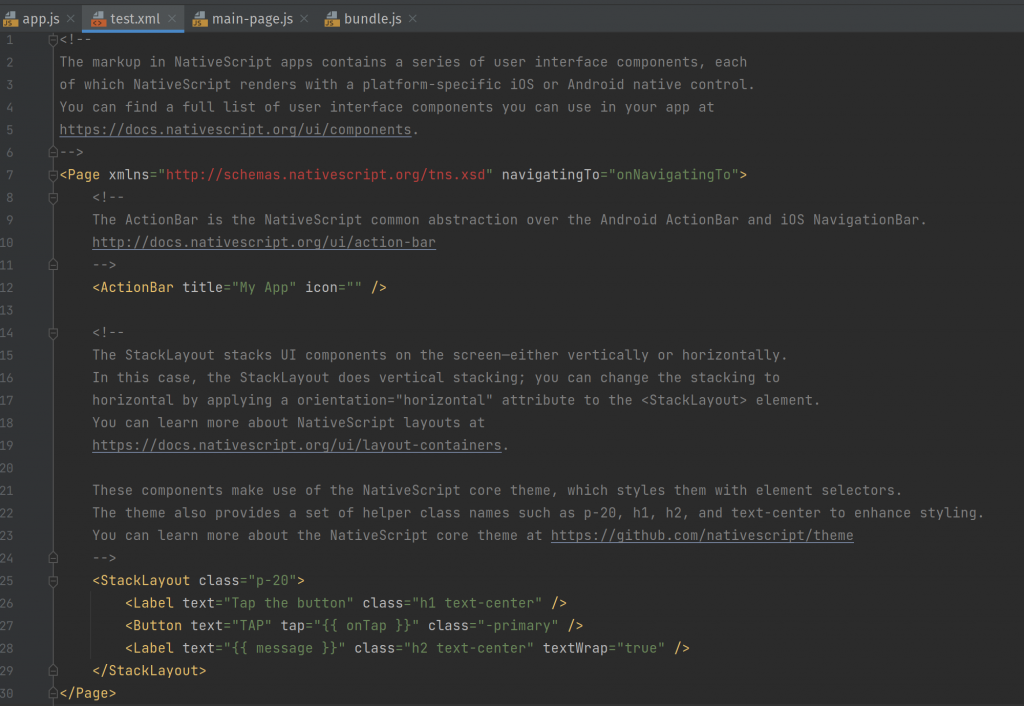
Uh oh...
Can anyone tell me how quickly I can take your fully released app, that you spent months or years on creating and make my own 100% full clone? Or perhaps even do things more nefarious and add, edit, or steal any data from any of your private endpoints?
What can you do about this?
There is three things that I'm aware of
- Minification (Built in to NativeScript & React Native)
- Obsfucation (Lots of different tools)
- Encryption
Minification
Well, the first thing people think of the minimization using Terser (or uglify). NativeScript uses Terser by default.
Lets see what happens when we minimize the code using NativeScript 8. Here is the bundle.js file using minification.
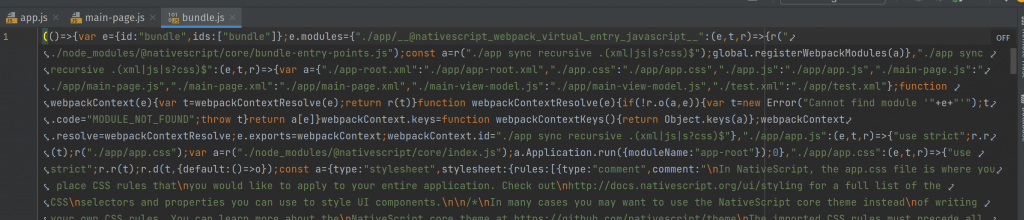
This looks hard to parse... But lets see what happens if we use the awesome "Reformat code" option in phpStorm/webStorm...
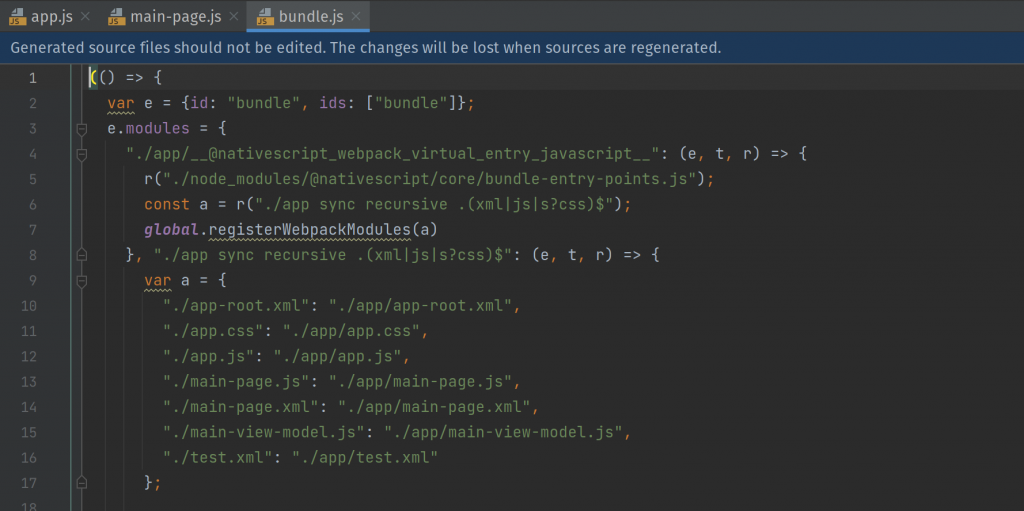
Well, it is different, but still totally readable. How about the main-page.js and our secret keys?
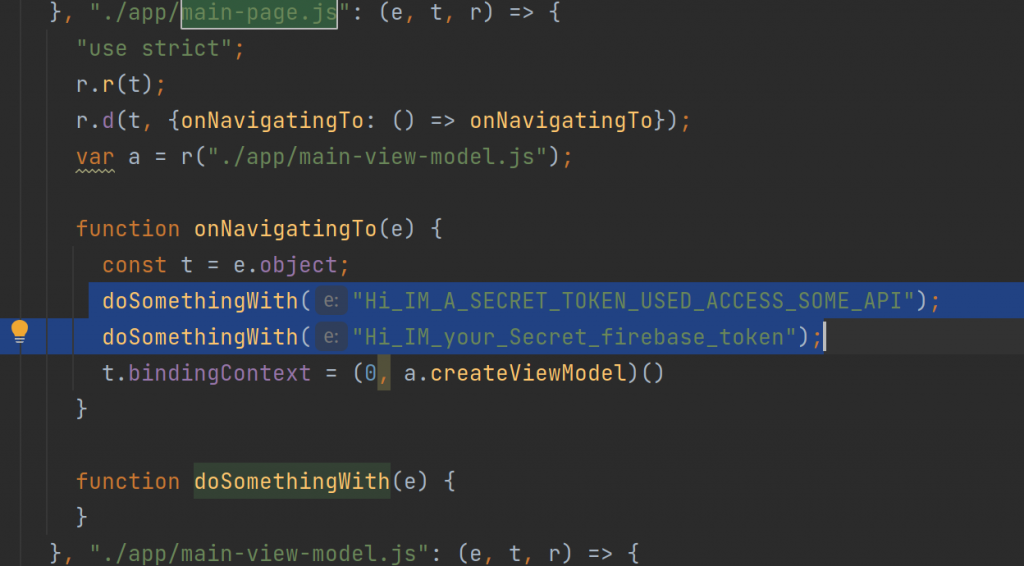
Well that id disappointing, the code is perfectly readable and usable. All your secrets api keys are well, not at all secret. The only thing missing is all the comments were stripped and my "console.log" was removed from the doSomethingWith routine. But the code is very readable and can be dropped virtually as-is in a new main-file.js javascript file. If I wanted to make this file to work in a new app, I would just need to replace "r" with "require" and add the "export" to the onNavigatingTo function and it would be 100% working. Add a couple refactors like "t" to "page" and extract the API keys to variables and it would be virtually identically to the original you wrote. Lets see, hacker 5 minutes, you 5 months.
As you can see minification does nothing really to hinder anyone. But it is a good step for optimization but adds zero security.
Obfuscation
What about obfuscation, this really depends on the tool. I have a blog post about this security of this. However, rather than re-iterate it, it is almost as trivial to reverse as we just did with minification. The only tool that took me longer than a few minutes to reverse was JSScrambler. However for JSScrambler to work you have to set this at its highest level and enable code protections so that a hacker can't easily modify the code. Unfortunately, the last time I tried this is slowed all of the code down by 30-50% the entire life time of the application.
Compiled Code
This is probably the next best thing, if you create a function that has your api keys encrypted in it and you have to call a couple functions to return them before you use them they won't be visible in the source which seems like an awesome win. However, if your JavaScript code has:
const api = getAPI(); const key = getKey; https.get(api+key);
Do you have any idea how quickly I'll get your api & key, I'll just change the code to be:
const api = getAPI(); const key = getKey; console.log(api+key);
Did you slow me down, yes, by how much -- maybe 15 seconds to modify the apk and upload it to by emulator (or a jail broken android/ios device) . So if you use this method, please do NOT telegraph how to get your Key & api endpoints. At least be more discrete as when I do a search for "https" or "firebase" in your code base you don't want to point me to where I need to go next.
React Native offers a engine called Hermes where it partially compiles the code, this is more secure than the pure JS code mode, but obviously not as secure as pure compiled. However, even in pure compiled code, if you do nothing to obfuscate your keys and api end points, just running strings against it will give you everything a hacker needs to attack your end points.
Encrypted Code
First a major disclaimer here -- I am the author of the AppProtection system, of course I recommend it. Second disclaimer, this is currently NativeScript only, but if I get enough interest for a React Native version, I'll port it as I have all the code all done for both v8 and JavaScriptCore engines. But I have to spend the time adding it to the React Native tooling. Basically this encrypts the source code for your release apps. This is what a release file looks like:
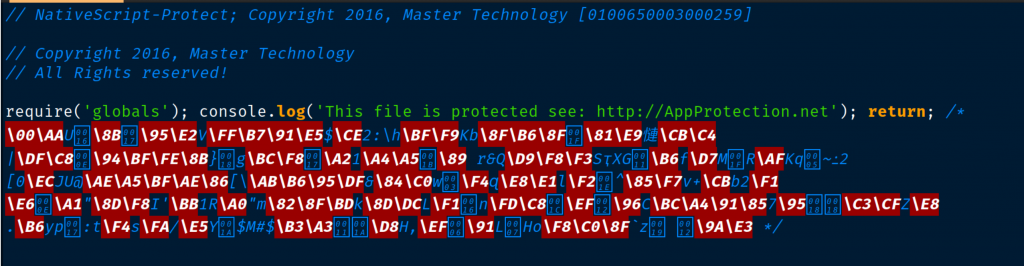
The source code AES encrypted, and the only unencrypted copy is in memory (never saved to disk) while the app is running. The actual v8/JSC runtime engine itself has the modifications for understanding AES encrypted JS code, no extra code is shipped in your app. Is it perfect? Nope, but it adds another very difficult wall to climb...
The 100% Solution
Click bait! Sorry there is no 100% solutions. I haven't heard of any of the AppProtection applications being cracked, but that doesn't mean it can't be (or hasn't been). I will tell you upfront everything can be cracked, including AppProtection. Even Denovo which is used in high profile games and has millions of dollars in revenue. Eventually, each new release gets cracked. Everything can be hacked with enough time, patience and knowledge. However, the whole idea of security is you put enough walls in front of your attacker to make him want to go to a much easier target. If they leave, then you have thwarted an attack. Do you really think most attackers or application cloners want to spend weeks pulling your data or cloning your app, or switch to something that only takes them a few minutes?
More information
- I have another blog post I wrote in 2019, everything is still valid and I discuss other things you can do in your app for security and other security gotcha's.
- I am available to do consulting work to help you beef up your security both server and mobile. I also do core reviews, and training if your developers need to learn some better practices.
- You can email me at nathan@master.technology
Nice